當(dāng)前文章介紹動(dòng)態(tài)堆空間內(nèi)存分配與釋放,C語(yǔ)言結(jié)構(gòu)體定義、初始化、賦值、結(jié)構(gòu)體數(shù)組、結(jié)構(gòu)體指針的相關(guān)知識(shí)點(diǎn),最后通過(guò)一個(gè)學(xué)生管理系統(tǒng)綜合練習(xí)結(jié)構(gòu)體數(shù)組的使用。
1. 動(dòng)態(tài)內(nèi)存管理
C語(yǔ)言代碼----->編譯----->鏈接------>可執(zhí)行的二進(jìn)制文件(windows下xxx.exe)
二進(jìn)制文件中的數(shù)據(jù)是如何擺放的? 文本數(shù)據(jù)段、靜態(tài)數(shù)據(jù)段、全局?jǐn)?shù)據(jù)段。
堆??臻g: 代碼在運(yùn)行的時(shí)候才有的空間。
棧空間: 系統(tǒng)負(fù)責(zé)申請(qǐng),負(fù)責(zé)釋放。比如: 函數(shù)形參變量、數(shù)組……
堆空間: 程序員負(fù)責(zé)申請(qǐng),負(fù)責(zé)釋放。
#include //標(biāo)準(zhǔn)庫(kù)頭文件
void *malloc(int size); //內(nèi)存申請(qǐng)。 形參表示申請(qǐng)的空間大小,返回值:申請(qǐng)的空間的地址
void free(void *p); //內(nèi)存釋放。 形參就是要釋放的空間首地址。
動(dòng)態(tài)空間申請(qǐng)示例。
動(dòng)態(tài)空間申請(qǐng)
#include "stdio.h"
#include "string.h"
#include
int main()
{
int *p=malloc(sizeof(int)); //申請(qǐng)空間
if(p!=NULL)
{
printf("申請(qǐng)的空間地址: 0x%X\n",p);
*p=888;
printf("%d\n",*p);
}
free(p); //釋放空間
return 0;
}
示例2:
#include "stdio.h"
#include "string.h"
#include
char *func(void)
{
char*str=malloc(100); //char str[100];
if(str!=NULL)
{
strcpy(str,"1234567890");
printf("子函數(shù)打印:%s\n",str);
//free(str); //釋放空間
return str;
}
else
{
return NULL;
}
}
int main()
{
char *p=func();
printf("主函數(shù)打印:%s\n",p);
return 0;
}
2. 結(jié)構(gòu)體
2.1 定義語(yǔ)法
結(jié)構(gòu)體的概念: 可存放不同數(shù)據(jù)類(lèi)型的集合。
比如: 存放一個(gè)班級(jí)學(xué)生的信息。
可以使用一個(gè)結(jié)構(gòu)體存放一個(gè)學(xué)生的信息。
一個(gè)結(jié)構(gòu)體數(shù)組存放整個(gè)班級(jí)的學(xué)習(xí)信息。
數(shù)組的概念: 可存放相同數(shù)據(jù)類(lèi)型的集合。
結(jié)構(gòu)體的定義語(yǔ)法:
//聲明一種新類(lèi)型-----數(shù)據(jù)類(lèi)型
struct <結(jié)構(gòu)體的名稱(chēng)>
{
<結(jié)構(gòu)體的成員>1;
<結(jié)構(gòu)體的成員>2;
…………
}; //最后有分號(hào)結(jié)束
struct MyStruct
{
char a;
int b;
float c;
char str[100];
};
2.2 定義示例
結(jié)構(gòu)體如何賦值? 如何訪問(wèn)結(jié)構(gòu)體內(nèi)部成員
#include "stdio.h"
#include "string.h"
#include
//定義結(jié)構(gòu)體數(shù)據(jù)類(lèi)型
struct MyStruct
{
char a;
int b;
float c;
char str[100];
};
int main()
{
struct MyStruct data={'A',123,456.789,"abcd"}; //data就是結(jié)構(gòu)體類(lèi)型的變量
//結(jié)構(gòu)體變量訪問(wèn)內(nèi)部成員的語(yǔ)法: . 點(diǎn)運(yùn)算符
printf("%c\n",data.a);
printf("%d\n",data.b);
printf("%f\n",data.c);
printf("%s\n",data.str);
return 0;
}
2.3 初始化
#include "stdio.h"
#include "string.h"
#include
//定義結(jié)構(gòu)體數(shù)據(jù)類(lèi)型
struct MyStruct
{
char a;
int b;
float c;
char str[100];
}data={'A',123,456.789,"abcd"}; //data就是結(jié)構(gòu)體類(lèi)型的變量
int main()
{
//結(jié)構(gòu)體變量訪問(wèn)內(nèi)部成員的語(yǔ)法: . 點(diǎn)運(yùn)算符
printf("%c\n",data.a);
printf("%d\n",data.b);
printf("%f\n",data.c);
printf("%s\n",data.str);
return 0;
}
2.4 結(jié)構(gòu)體賦值
//結(jié)構(gòu)體變量訪問(wèn)內(nèi)部成員的語(yǔ)法: . 點(diǎn)運(yùn)算符
#include "stdio.h"
#include "string.h"
#include
//定義結(jié)構(gòu)體數(shù)據(jù)類(lèi)型
struct MyStruct
{
char a;
int b;
float c;
char str[100];
};
int main()
{
struct MyStruct data;//data就是結(jié)構(gòu)體類(lèi)型的變量
//成員單獨(dú)賦值
data.a='A';
data.b=123;
data.c=456.789;
strcpy(data.str,"abcd"); //數(shù)組賦值
//結(jié)構(gòu)體變量訪問(wèn)內(nèi)部成員的語(yǔ)法: . 點(diǎn)運(yùn)算符
printf("%c\n",data.a);
printf("%d\n",data.b);
printf("%f\n",data.c);
printf("%s\n",data.str);
return 0;
}
2.5 結(jié)構(gòu)體數(shù)組
結(jié)構(gòu)體賦值分為兩種標(biāo)準(zhǔn): C89 、C99
?結(jié)構(gòu)體數(shù)組
#include "stdio.h"
#include "string.h"
#include
//定義結(jié)構(gòu)體數(shù)據(jù)類(lèi)型
struct MyStruct
{
char a;
int b;
float c;
char str[100];
};
int main()
{
struct MyStruct data[100];//data就是結(jié)構(gòu)體數(shù)組類(lèi)型變量
struct MyStruct data2[50];
//成員單獨(dú)賦值
data[0].a='A';
data[0].b=123;
data[0].c=456.789;
strcpy(data[0].str,"abcd"); //數(shù)組賦值
//結(jié)構(gòu)體變量訪問(wèn)內(nèi)部成員的語(yǔ)法: . 點(diǎn)運(yùn)算符
printf("%c\n",data[0].a);
printf("%d\n",data[0].b);
printf("%f\n",data[0].c);
printf("%s\n",data[0].str);
return 0;
}
2.6 結(jié)構(gòu)體指針賦值
#include "stdio.h"
#include "string.h"
#include
//定義結(jié)構(gòu)體數(shù)據(jù)類(lèi)型
struct MyStruct
{
char a;
int b;
float c;
char str[100];
};
int main()
{
//struct MyStruct buff[100];
//struct MyStruct *data=buff; //結(jié)構(gòu)體指針類(lèi)型變量
struct MyStruct *data=malloc(sizeof(struct MyStruct));
data->a='A';
data->b=123;
data->c=456.789;
strcpy(data->str,"abcd");
//結(jié)構(gòu)體指針訪問(wèn)內(nèi)部成員的變量 通過(guò) -> 運(yùn)算符。
printf("%c\n",data->a);
printf("%d\n",data->b);
printf("%f\n",data->c);
printf("%s\n",data->str);
return 0;
}
3. 學(xué)生管理系統(tǒng)
作業(yè): 學(xué)生管理系統(tǒng)
需求: (每一個(gè)功能都是使用函數(shù)進(jìn)行封裝)
1.實(shí)現(xiàn)從鍵盤(pán)上錄入學(xué)生信息。 (姓名、性別、學(xué)號(hào)、成績(jī)、電話(huà)號(hào)碼)
2.將結(jié)構(gòu)體里的學(xué)生信息全部打印出來(lái)。
3.實(shí)現(xiàn)根據(jù)學(xué)生的姓名或者學(xué)號(hào)查找學(xué)生,查找到之后打印出學(xué)生的具體信息。
4.根據(jù)學(xué)生的成績(jī)對(duì)學(xué)生信息進(jìn)行排序。
5.根據(jù)學(xué)號(hào)刪除學(xué)生信息。
示例:
#include "stdio.h"
#include "string.h"
#include
//定義存放學(xué)生信息的結(jié)構(gòu)體類(lèi)型
struct StuDentInfo
{
char Name[20]; //姓名
int number; //學(xué)號(hào)
char phone[20];//電話(huà)號(hào)碼
};
//全局變量區(qū)域
unsigned int StuDentCnt=0; //記錄已經(jīng)錄入的全部學(xué)生數(shù)量
//函數(shù)聲明區(qū)域
void PrintStuDentInfoList(void);
void InputStuDentInfo(struct StuDentInfo*info);
void FindStuDentInfo(struct StuDentInfo*info);
void SortStuDentInfo(struct StuDentInfo*info);
void PrintStuDentInfo(struct StuDentInfo*info);
int main()
{
struct StuDentInfo data[100]; //可以100位學(xué)生的信息
int number;
while(1)
{
PrintStuDentInfoList(); //打印功能列表
scanf("%d",&number);
printf("\n");
switch(number)
{
case 1:
InputStuDentInfo(data);
break;
case 2:
FindStuDentInfo(data);
break;
case 3:
SortStuDentInfo(data);
break;
case 4:
PrintStuDentInfo(data);
break;
case 5:
break;
default:
printf("選擇錯(cuò)誤!\n\n");
break;
}
}
return 0;
}
/*
函數(shù)功能: 打印學(xué)生管理系統(tǒng)的功能列表
*/
void PrintStuDentInfoList(void)
{
printf("\n--------------學(xué)生管理系統(tǒng)功能列表----------------\n");
printf("1. 錄入學(xué)生信息\n");
printf("2. 根據(jù)學(xué)號(hào)查找學(xué)生信息\n");
printf("3. 根據(jù)學(xué)號(hào)排序\n");
printf("4. 打印所有學(xué)生信息\n");
printf("5. 刪除指定的學(xué)生信息\n");
printf("請(qǐng)選擇功能序號(hào):");
}
/*
函數(shù)功能: 錄入學(xué)生信息
*/
void InputStuDentInfo(struct StuDentInfo*info)
{
printf("輸入學(xué)生姓名:");
scanf("%s",info[StuDentCnt].Name);
printf("輸入學(xué)號(hào):");
scanf("%d",&info[StuDentCnt].number);
printf("輸入電話(huà)號(hào)碼:");
scanf("%s",info[StuDentCnt].phone);
StuDentCnt++; //數(shù)量自增
}
/*
函數(shù)功能: 查找學(xué)生信息
*/
void FindStuDentInfo(struct StuDentInfo*info)
{
int num,i;
printf("輸入查找的學(xué)號(hào):");
scanf("%d",&num);
for(i=0; iinfo[j+1].number)
{
tmp=info[j];
info[j]=info[j+1];
info[j+1]=tmp;
}
}
}
}
/*
函數(shù)功能: 打印所有學(xué)生信息
*/
void PrintStuDentInfo(struct StuDentInfo*info)
{
int i=0;
printf("-----------所有學(xué)生的信息列表------------\n");
for(i=0;i
;i++)>;>
-
C語(yǔ)言
+關(guān)注
關(guān)注
180文章
7614瀏覽量
137800 -
結(jié)構(gòu)體
+關(guān)注
關(guān)注
1文章
130瀏覽量
10872
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
嵌入式C語(yǔ)言知識(shí)點(diǎn)總結(jié)
漫談C語(yǔ)言結(jié)構(gòu)體
dxp整體總結(jié)
C語(yǔ)言字符串操作總結(jié)大全(超詳細(xì))
C語(yǔ)言入門(mén)教程之順序結(jié)構(gòu)總結(jié)的詳細(xì)資料概述
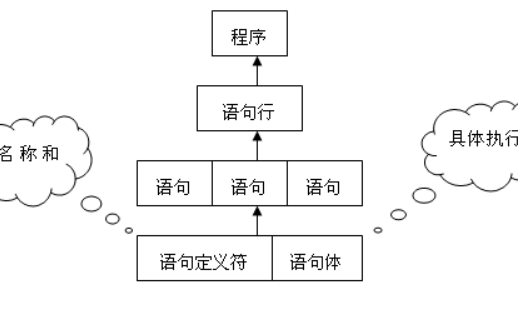
C語(yǔ)言入門(mén)教程之循環(huán)結(jié)構(gòu)總結(jié)的詳細(xì)資料概述
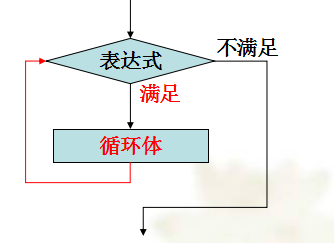
適合C語(yǔ)言小白看的基礎(chǔ)知識(shí)梳理總結(jié)
C語(yǔ)言教程之C基礎(chǔ)變量的技術(shù)總結(jié)
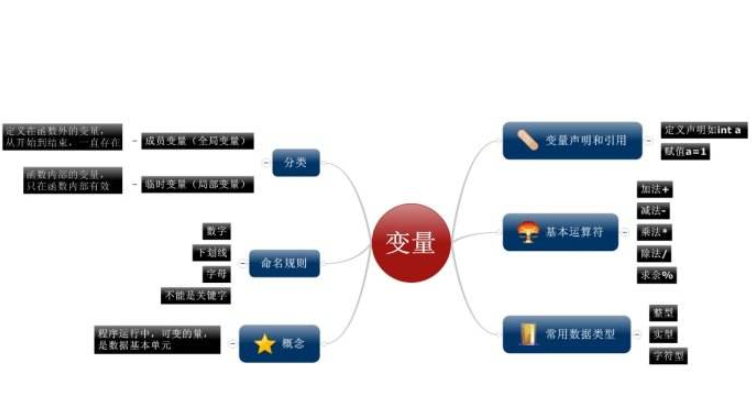
C語(yǔ)言的拓展歸納總結(jié)詳細(xì)說(shuō)明
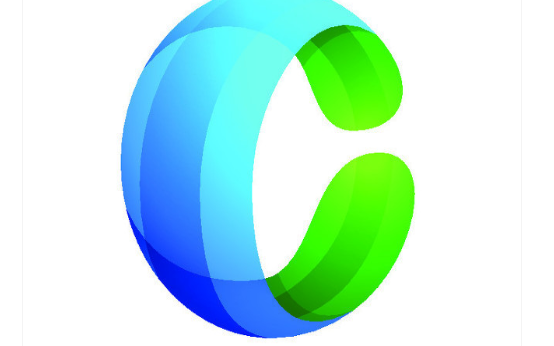
嵌入式C語(yǔ)言知識(shí)總結(jié)
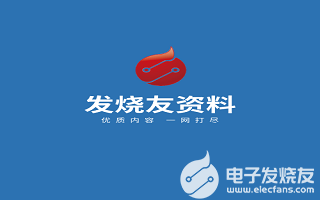
評(píng)論